How to add a gift wrapper programmatically. The following method supports WPML plugin and for the style is using the classes of the DIVI theme.
1) Add a product “Gift Wrap” and get the ID. In case of having more than one languages with WPML there are going to be one ID per language.
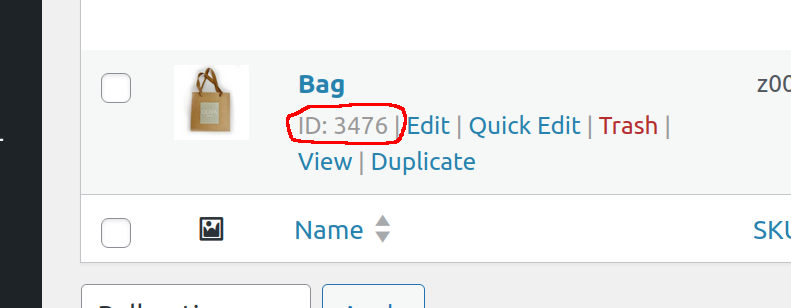
2) Edit the function.php file of your theme. Add the following code:
function is_in_cart( $ids )
{
// Initialise
$found = false;
// Loop through cart items
foreach( WC()->cart->get_cart() as $cart_item ) {
// For an array of product IDs
if( is_array($ids) && ( in_array( $cart_item['product_id'], $ids ) || in_array( $cart_item['variation_id'], $ids ) ) ){
$found = true;
break;
}
// For a unique product ID (integer or string value)
elseif( ! is_array($ids) && ( $ids == $cart_item['product_id'] || $ids == $cart_item['variation_id'] ) ){
$found = true;
break;
}
}
return $found;
}
function my_add_gift_wrap()
{
$product_id_el = 3475;
$product_id_en = 3476;
// show the link to add the gift wrap product, only, if is not already added
if( !(is_in_cart($product_id_el) || is_in_cart($product_id_en)) )
{
if(ICL_LANGUAGE_CODE=='en') // WPML if the language is in English
{
echo "<a id='sms_add_gift_wrap_button' style='color: #FFFFFF !important; margin-bottom: 10px;' class='button et_pb_contact_submit et_pb_button' href='https://your_domain.com/en/cart/?add-to-cart=$product_id_en'>Add Gift Wrapping $in_cart</a>";
}
else
{
echo "<a id='sms_add_gift_wrap_button' style='color: #FFFFFF !important; margin-bottom: 10px;' class='button et_pb_contact_submit et_pb_button' href='https://your_domain.com/cart/?add-to-cart=$product_id_el'>Add Gift Wrapping In Other Language </a>";
}
}
}
add_action('woocommerce_after_cart_contents', 'my_add_gift_wrap');
{
// Initialise
$found = false;
// Loop through cart items
foreach( WC()->cart->get_cart() as $cart_item ) {
// For an array of product IDs
if( is_array($ids) && ( in_array( $cart_item['product_id'], $ids ) || in_array( $cart_item['variation_id'], $ids ) ) ){
$found = true;
break;
}
// For a unique product ID (integer or string value)
elseif( ! is_array($ids) && ( $ids == $cart_item['product_id'] || $ids == $cart_item['variation_id'] ) ){
$found = true;
break;
}
}
return $found;
}
function my_add_gift_wrap()
{
$product_id_el = 3475;
$product_id_en = 3476;
// show the link to add the gift wrap product, only, if is not already added
if( !(is_in_cart($product_id_el) || is_in_cart($product_id_en)) )
{
if(ICL_LANGUAGE_CODE=='en') // WPML if the language is in English
{
echo "<a id='sms_add_gift_wrap_button' style='color: #FFFFFF !important; margin-bottom: 10px;' class='button et_pb_contact_submit et_pb_button' href='https://your_domain.com/en/cart/?add-to-cart=$product_id_en'>Add Gift Wrapping $in_cart</a>";
}
else
{
echo "<a id='sms_add_gift_wrap_button' style='color: #FFFFFF !important; margin-bottom: 10px;' class='button et_pb_contact_submit et_pb_button' href='https://your_domain.com/cart/?add-to-cart=$product_id_el'>Add Gift Wrapping In Other Language </a>";
}
}
}
add_action('woocommerce_after_cart_contents', 'my_add_gift_wrap');
Reference to directly add a product in your cart:
https://usersinsights.com/add-to-cart-url-woocommerce/
Reference of the function is_in_cart:
https://stackoverflow.com/questions/49934274/woocommerce-if-condition-if-product-is-in-cart-do-something
Reference to add something in your woocommerce cart:
https://stackoverflow.com/questions/27810529/woocommerce-add-function-to-run-after-display-items-in-cart